Unit Testing with ChatGPT
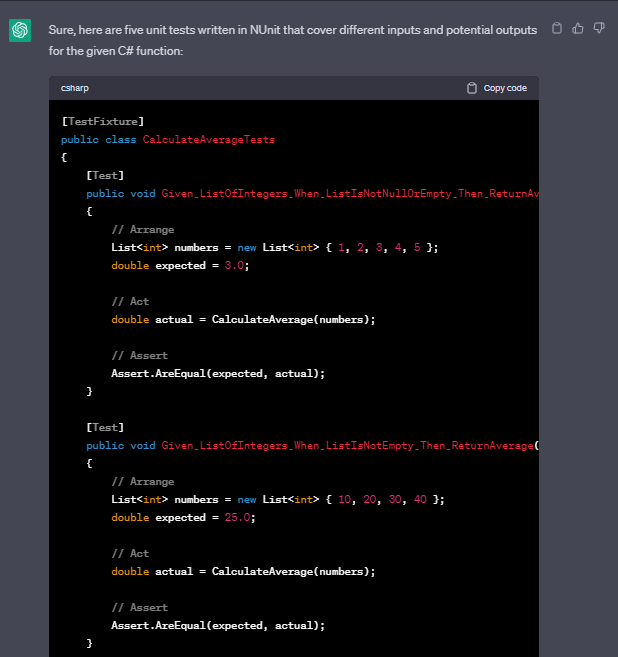
Don't like writing unit tests or simply don't have the time for it? Good news – now you don't have to! As a newbord AI enthusiast with a long-time obsession with unit testing, I couldn't resist the urge to try and combine these two topics. Check out my latest blog post "Unit Testing with ChatGPT," to discover how you can use ChatGPT to generate test cases and code for you.…